Referencing System.Windows.Controls.dll
Before we start using the TreeView control we need to add a reference to
System.Windows.Controls.dll
. This assembly can be found in the "Microsoft Silverlight 4 Tools for Visual Studio 2010" package.You need to copy this assembly to the location of
chiron.exe
(ex. IronPython2.7\Silverlight\bin
) and add a reference to it in the AppManifest.xaml
file.<Deployment xmlns="http://schemas.microsoft.com/client/2007/deployment"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
RuntimeVersion="4.0.50917.0"
EntryPointAssembly="Microsoft.Scripting.Silverlight"
EntryPointType="Microsoft.Scripting.Silverlight.DynamicApplication"
ExternalCallersFromCrossDomain="ScriptableOnly">
<!-- Add assembly references here -->
<Deployment.Parts>
...
<AssemblyPart Source="System.Windows.Controls.dll" />
</Deployment.Parts>
...
</Deployment>
Since we're using Silverlight 4 we need to change the runtime version to "4.0.50917.0" (see this post for more details).
Using the TreeView in XAML
Now that we have access to the assembly we can use it in
app.xaml
.<UserControl x:Class="System.Windows.Controls.UserControl"
xmlns="http://schemas.microsoft.com/client/2007"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:sdk="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls"
xmlns:wsdk="clr-namespace:System.Windows;assembly=System.Windows.Controls">
<StackPanel x:Name="layout_root" Background="White">
<TextBlock x:Name="Message" FontSize="30" Text="TreeView experiment" />
<sdk:TreeView x:Name="tree">
<sdk:TreeView.ItemTemplate>
<wsdk:HierarchicalDataTemplate ItemsSource="{Binding nodes}">
<TextBlock Foreground="Red" Text="{Binding label}" />
</wsdk:HierarchicalDataTemplate>
</sdk:TreeView.ItemTemplate>
</sdk:TreeView>
</StackPanel>
</UserControl>
TreeView data binding
The XAML file above shows that we're binding the content of the tree view to the
nodes
property of the data source object. Here's the definition of the Python class used for this example:from System.Windows import Application
from System.Windows.Controls import UserControl
from System import Object
from System.Collections.ObjectModel import ObservableCollection
import clrtype
import clr
clr.AddReferenceToFile('GalaSoft.MvvmLight.SL4.dll')
from GalaSoft.MvvmLight.Command import RelayCommand
class Node:
__metaclass__ = clrtype.ClrClass
__clrnamespace = "LangexplrExperiments"
def __init__(self,label,children):
self.text = label
self.children = children
@property
@clrtype.accepts()
@clrtype.returns(str)
def label(self):
return self.text
@property
@clrtype.accepts()
@clrtype.returns(Object)
def nodes(self):
return self.children
class App:
def __init__(self):
root = Application.Current.LoadRootVisual(UserControl(), "app.xaml")
root.tree.ItemsSource = [Node('level1',
[Node('level11',[]),
Node('level12',[Node('level121',[])])
])]
App()
With this changes we can run
chiron.exe /b
and we get: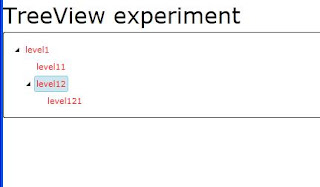